Available Functions and Distributions
Output Distributions
The distributions are provided by Scipy’s stats module. Instead of calling the .rvs()
method, however, we use the .ppf()
(percent point function) method to obtain realizations given the error terms.
For the parameters with described range, we can specify correction.
Distribution |
Parameters |
Range |
---|---|---|
|
|
\([0, 1]\) |
|
|
\(\mathbb{R}\) |
|
\(\mathbb{R}\) |
|
|
|
\(\mathbb{R}\) |
|
\(\mathbb{R}_+\) |
|
|
|
\(\mathbb{R}\) |
|
\(\mathbb{R}_+\) |
|
|
|
\(\mathbb{N}_0\) |
|
|
\(\mathbb{R}_+\) |
Edge Functions
Read more about each edge function by clicking the link in the table title.
Identity
Formula
PARCS Code
pyparcs.api.mapping_functions.py
1 return array
2
3
Parameters
identity()
edge function has no parameters.
Sigmoid
Formula
PARCS Code
pyparcs.api.mapping_functions.py
1 """Sigmoid edge function"""
2 parcs_assert(gamma in [0, 1] and tau % 2 == 1,
3 EdgeFunctionError,
4 f'error in gamma={gamma} or tau={tau} values')
5
6 expon = (-1) ** gamma * alpha * ((array - beta) ** tau)
7 return expit(expon)
8
9
10def edge_gaussian_rbf(array: np.ndarray,
Parameters
Parameter |
Description |
Default |
---|---|---|
\(\alpha\): |
Scale of the Sigmoid. Takes positive values.
The reasonable range is approx. \([1, 3]\).
|
|
\(\beta\): |
Offset of the Sigmoid. Takes real values.
The reasonable range is approx. \([-2, 2]\).
|
|
\(\gamma\): |
Mirroring on x-axis. Takes \(\{0, 1\}\). |
|
\(\tau\): |
Induces a dwelling region in the center of the function.
Takes odd values \(\{1, 3, \dots\}\).
|
|
The figures below depict the effect of each parameter on the sigmoid transformation:
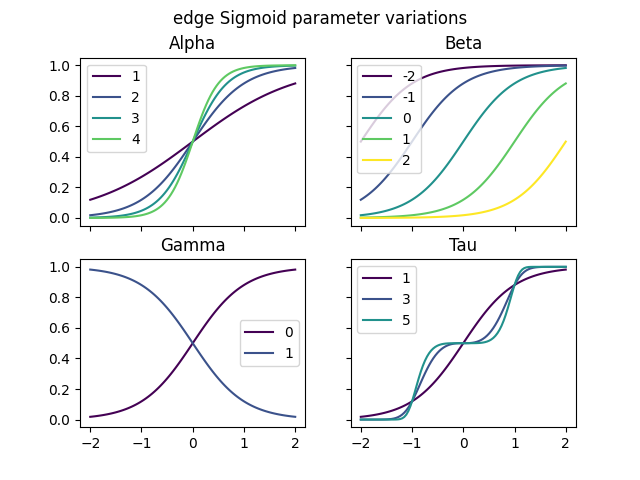
Gaussian RBF
Formula
PARCS Code
pyparcs.api.mapping_functions.py
1 parcs_assert(gamma in [0, 1] and tau % 2 == 0,
2 EdgeFunctionError,
3 f'error in gamma={gamma} or tau={tau} values')
4
5 expon = -alpha * ((array - beta) ** tau)
6 return gamma + ((-1) ** gamma) * np.exp(expon)
7
8
9def edge_arctan(array: np.ndarray,
10 alpha: float = 2, beta: float = 0, gamma: float = 0) -> np.ndarray:
Parameters
Parameter |
Description |
Default |
---|---|---|
\(\alpha\): |
Scale of the RBF. Takes positive values.
The reasonable range is approx. \([1, 3]\).
|
|
\(\beta\): |
Offset of the RBF. Takes real values.
The reasonable range is approx. \([-2, 2]\).
|
|
\(\gamma\): |
Mirroring on y-axis. Takes \(\{0, 1\}\). |
|
\(\tau\): |
Induces a dwelling region in the center of the function.
Takes even values \(\{2, 4, \dots\}\).
|
|
The figures below depict the effect of each parameter on the Gaussian RBF transformation:
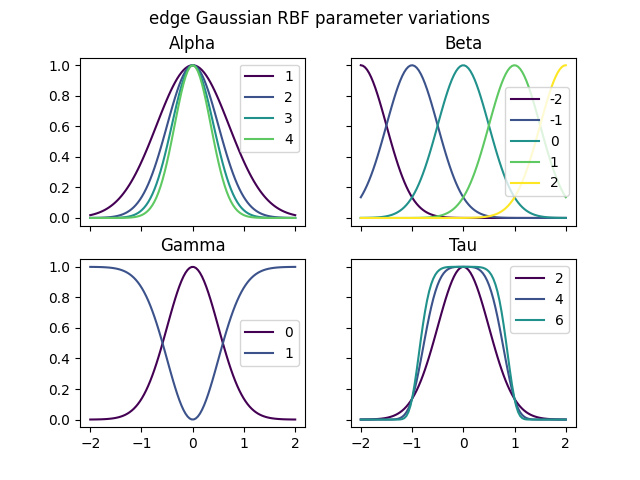
Arctan
Formula
PARCS Code
pyparcs.api.mapping_functions.py
1 f'error in gamma={gamma} value')
2
3 return (-1) ** gamma * np.arctan(alpha * (array - beta))
4
5
6EDGE_FUNCTIONS = {
7 'identity': edge_identity,
8 'sigmoid': edge_sigmoid,
Parameters
Parameter |
Description |
Default |
---|---|---|
\(\alpha\): |
Scale of the RBF. Takes positive values.
The reasonable range is approx. \([1, 3]\)
|
|
\(\beta\): |
Offset of the RBF. Takes real values.
The reasonable range is approx. \([-2, 2]\)
|
|
\(\gamma\): |
Mirroring on y-axis. Takes \(\{0, 1\}\) |
|
The figures below depict the effect of each parameter on the arctan transformation:
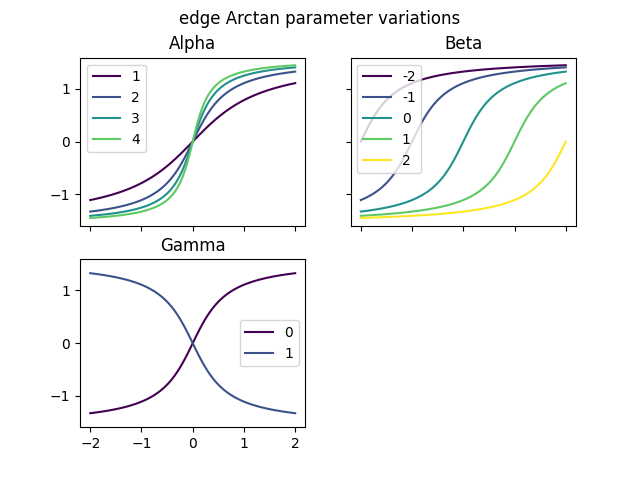
Corrections
Sigmoid Correction
Parameter |
Description |
type |
default (yml/py) |
---|---|---|---|
|
Lower and upper range of the corrected values. |
float |
0, 1 |
|
If the raw values must be centered (mean = 0)
before sigmoid correction.
|
bool |
false/False |
|
Specify this option if you want to fix the mean
of corrected values. Must be in range of (lower, upper)
|
float |
null/None |
Edge Correction
Parameter |
Description |
type |
default (yml/py) |
---|---|---|---|